1. LeanXcale NoSQL Database Integration with Hibernate ORM
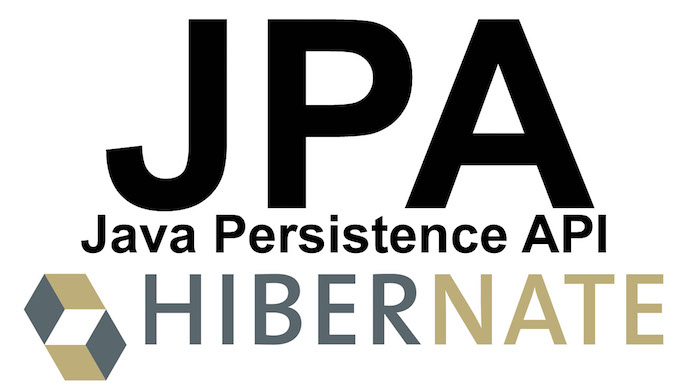
Hibernate ORM is a robust Object-Relational Mapping (ORM) framework tailored for Java applications. Its core strength lies in ensuring efficient data persistence for relational databases.
The LeanXcale database seamlessly integrates with Hibernate ORM for Java applications, offering robust data persistence capabilities. This guide will walk you through getting started with Hibernate ORM for LeanXcale.
1.1. Prerequisites
The application using Hibernate ORM on LeanXcale should meet the following prerequisites:
-
Java Development Kit (JDK) 11 or later is installed.
-
Use Apache Maven Apache Maven 3.3 or later.
-
Use Hibernate 6.0 or later.
1.2. Create a CRUD with Hibernate and LeanXcale
Discover how to connect to your LeanXcale database and initiate fundamental CRUD operations by following the instructions on the Java ORM example application page. Subsequent sections will illustrate the essential procedures for Java application development employing Hibernate ORM while integrating seamlessly with LeanXcale.
1.2.1. Configure the Hibernate ORM Dependency
To use the Hibernate connector/dialect, include the dependency in your Maven configuration:
REVISAR
<dependency>
<groupId>com.leanxcale.connectors</groupId>
<artifactId>hibernate-connector</artifactId>
<version>2.0.0</version>
</dependency>
Be sure to include the LeanXcale public Maven repository in your Maven configuration:
<repositories>
<repository>
<id>lx-public</id>
<url>https://artifactory.leanxcale.com/artifactory/lx-public/</url>
</repository>
</repositories>
If you don’t use Maven or prefer to include the libraries in your project manually, download the Hibernate Connector from the download page.
1.2.2. Map the Hibernate ORM
Create a class named Payment and use the following code. Please note that we will use Lombok @Data notation to simplify the definition
package com.leanxcale.cm.payment;
import jakarta.persistence.Entity;
import jakarta.persistence.Id;
import jakarta.persistence.Table;
import lombok.Data;
@Data
@Entity
@Table(name = "payment")
public class Payment {
@Id
private Integer id;
private String customer;
private double amount;
}
1.2.3. Create a DAO Object for the Company Object
Establish a Data Access Object (DAO) named PaymentDAO.java. This DAO is the implementation hub for fundamental CRUD operations involving the Payment.java domain object. You can incorporate the provided sample code into your project for seamless integration with LeanXcale.
package com.leanxcale.cm.payment;
import java.util.Optional;
import org.hibernate.Session;
import org.hibernate.Transaction;
public class PaymentDao { //FIXME delete!
Session hibernateSession;
public PaymentDao (Session session) {
hibernateSession = session;
}
public void save(final Payment entity) {
Transaction transaction = hibernateSession.beginTransaction();
try {
hibernateSession.save(entity);
transaction.commit();
} catch(RuntimeException rte) {
transaction.rollback();
}
}
public Optional<Payment> findById(final Integer id) {
return Optional.ofNullable(hibernateSession.get(Payment.class, id));
}
public void delete(final Payment entity) {
Transaction transaction = hibernateSession.beginTransaction();
try {
hibernateSession.remove(entity);
transaction.commit();
} catch(RuntimeException rte) {
transaction.rollback();
}
}
public void update(final Payment entity) {
Transaction transaction = hibernateSession.beginTransaction();
try {
hibernateSession.merge(entity);
transaction.commit();
} catch(RuntimeException rte) {
transaction.rollback();
}
}
public void close(){
if (hibernateSession.isOpen()){
hibernateSession.close();
}
}
}
1.2.4. Set Hibernate Properties
Add the Hibernate configurations file hibernate.cfg.xml to the resources directory, and set up the following options.
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">com.leanxcale.client.Driver</property>
<property name="hibernate.connection.url">jdbc:leanxcale://localhost:14440/db;distribute=no</property>
<property name="hibernate.connection.username">APP</property>
<property name="hibernate.connection.password">APP</property>
<property name="hibernate.dialect">com.leanxcale.connector.hibernate.LxDialect</property>
<property name="show_sql">true</property>
<property name="hbm2ddl.auto">update</property>
<property name="hibernate.current_session_context_class">thread</property>
<property name="hibernate.globally_quoted_identifiers">true</property>
<mapping class="com.leanxcale.cm.payment.Payment"></mapping>
</session-factory>
</hibernate-configuration>
HIBERNATE PARAMETER | DESCRIPTION | DEFAULT |
---|---|---|
hibernate.dialect |
Dialect to use to generate SQL optimized for a particular database |
com.leanxcale.connector.hibernate.LxDialect |
hibernate.connection.driver_class |
JDBC Driver name |
com.leanxcale.client.Driver |
hibernate.connection.url |
JDBC Connection URL |
jdbc:leanxcale://localhost:1530/db;distribute=no |
hibernate.connection.username |
Username |
APP |
hibernate.connection.password |
Password |
APP |
Hibernate offers a comprehensive list of configuration properties that allows you to fine-tune various aspects and functionalities supported by the ORM. Please check the official Hibernate documentation.for more in-depth information and specific configurations.
1.2.5. Add the Object-Relational Mapping
Add a mapping for the Payment class in the hibernate.cfg.xml configuration file.
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-configuration SYSTEM
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
...
<mapping class="com.leanxcale.model.Payment"/>
</session-factory>
</hibernate-configuration>
1.2.6. To Query LeanXcale Database
Create a new class named QuickStartWithHibernate.java. Paste the provided sample code to execute queries on the table contents from your Java client using Hibernate ORM while connecting to your LeanXcale instance.
package com.leanxcale.cm.payment;
import java.sql.SQLException;
import java.util.Optional;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.boot.Metadata;
import org.hibernate.boot.MetadataSources;
import org.hibernate.boot.registry.StandardServiceRegistry;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
public class QuickStartWithHibernate {
public static void main(String[] args) {
SessionFactory sessionFactory = getSessionFactory();
Session session = sessionFactory.openSession();
System.out.println("Connected to the Leanxcale successfully.");
PaymentDao paymentDao = new PaymentDao(session);
// Save an payment
Payment payment = new Payment();
payment.setId(1);
payment.setCustomer("Acme");
payment.setAmount(123.45);
paymentDao.save(payment);
// Find the Payment
Optional<Payment> byId = paymentDao.findById(1);
if (byId.isPresent()) {
System.out.println("Got Payment:" + payment.toString());
}else {
System.out.println("Payment not found:" + 1);
}
session.close();
}
private static SessionFactory getSessionFactory() {
StandardServiceRegistry standardRegistry = new StandardServiceRegistryBuilder().configure().build();
Metadata metaData = new MetadataSources(standardRegistry).getMetadataBuilder().build();
return metaData.getSessionFactoryBuilder().build();
}
}
When you execute it, the application should output this:
2023-09-05 19:41:48,258 [main] INFO org.hibernate.orm.connections.access: HHH10001501: Connection obtained from JdbcConnectionAccess [org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator$ConnectionProviderJdbcConnectionAccess@5d2e6f62] for (non-JTA) DDL execution was not in auto-commit mode; the Connection 'local transaction' will be committed and the Connection will be set into auto-commit mode.
Hibernate: create table "payment" ("id" integer not null, "amount" double not null, "customer" varchar(255), primary key ("id"))
Connected to the Leanxcale successfully.
Hibernate: insert into "payment" ("amount","customer","id") values (?,?,?)
Got Payment:Payment(id=1, customer=Acme, amount=123.45)